728x90
flutter 커뮤니티에서 질문이 와서 GetxService로
RemoteConfig 구현하는 것 공유하게 되었다.
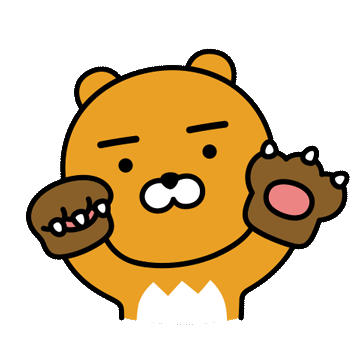
관리 중인 remote Config
아래의 코드
import 'dart:convert';
import 'dart:io';
import 'package:firebase_remote_config/firebase_remote_config.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:get/get.dart';
import 'package:matjipmemo/tools/logger.dart';
import 'package:package_info_plus/package_info_plus.dart';
import 'package:url_launcher/url_launcher.dart';
import '../models/firebase/point_give_model.dart';
class RemoteConfigService extends GetxService {
static String KEY_publish = 'publish';
static String KEY_necessary = 'necessary';
static String KEY_title = 'title';
static String KEY_points = 'points';
static String KEY_kill_app = 'kill_app';
static String KEY_contents = 'contents';
static String KEY_lastest_version_AOS = 'latest_version_AOS';
static String KEY_lastest_version_IOS = 'latest_version_IOS';
static String KEY_last_allow_build_version = 'last_allow_build_version';
static String KEY_notice_update = 'notice_update';
static String KEY_urgent_inspection_notice = 'urgent_inspection_notice';
static String androidStoreUrl =
'https://play.google.com/store/apps/details?id=kr.wagu.matjipmemo';
static String iosStoreUrl =
'https://apps.apple.com/us/app/eatrip/id1588490513';
final RxString _latestVersion = '1.2.6'.obs;
String get latestVersion => _latestVersion.value;
num lastAllowBuildVersion = 1; //이걸 높일수록 사용자가 작아짐
Map<String, dynamic> noticeUpdate = {};
Map<String, dynamic> noticeUrgentInspection = {};
late PointGiveModel points;
late PackageInfo packageInfo;
@override
void onInit() {
super.onInit();
readRemoteConfig();
}
Future readRemoteConfig() async {
logger.d('on readRemoteConfig');
packageInfo = await PackageInfo.fromPlatform();
final FirebaseRemoteConfig remoteConfig = FirebaseRemoteConfig.instance;
remoteConfig.setConfigSettings(RemoteConfigSettings(
fetchTimeout: const Duration(seconds: 10),
minimumFetchInterval: const Duration(minutes: 5),
));
remoteConfig.setDefaults({
KEY_last_allow_build_version: 1,
KEY_notice_update: '{}',
KEY_urgent_inspection_notice: '{}',
KEY_lastest_version_AOS: '1.0.0',
KEY_lastest_version_IOS: '1.0.0',
KEY_points: '{}',
});
logger.d('package buildNumber => ${packageInfo.buildNumber}\n'
'package name => ${packageInfo.packageName}'
'package appName => ${packageInfo.appName}'
'package version => ${packageInfo.version}');
bool update = await remoteConfig.fetchAndActivate();
if (update) {
logger.d('remote config update 됐다!!');
} else {
logger.d('remote config update 안 됐다!!');
}
_latestVersion.value = (!Platform.isIOS)
? remoteConfig.getString(KEY_lastest_version_AOS)
: remoteConfig.getString(KEY_lastest_version_IOS);
lastAllowBuildVersion = remoteConfig.getInt(KEY_last_allow_build_version);
noticeUpdate = json.decode(remoteConfig.getString(KEY_notice_update));
noticeUrgentInspection =
json.decode(remoteConfig.getString(KEY_urgent_inspection_notice));
points = PointGiveModel.fromJson(
json.decode(remoteConfig.getString(KEY_points)));
logger.d('my point => $points');
logger.d('official final build version => $lastAllowBuildVersion');
logger.d('noticeUpdate => $noticeUpdate');
logger.d(
'my dialog ${noticeUpdate[KEY_publish]} , ${lastAllowBuildVersion > int.parse(packageInfo.buildNumber)} '
', $lastAllowBuildVersion, ${int.parse(packageInfo.buildNumber)} ');
}
Future showRemoteConfigDialogs() async {
if ((noticeUpdate[KEY_publish] ?? false) &&
lastAllowBuildVersion > int.parse(packageInfo.buildNumber)) {
await updateDialog(noticeUpdate, packageInfo, latestVersion);
//
}
if (noticeUrgentInspection[KEY_publish] ?? false) {
await urgentDialog(noticeUrgentInspection, packageInfo, latestVersion);
//
}
}
Future<void> updateDialog(Map<String, dynamic> noticeUpdate,
PackageInfo packageInfo, String latestVersion) async {
bool isNecessary = noticeUpdate[KEY_necessary] ?? false;
Get.dialog(
WillPopScope(
onWillPop: () async {
return !isNecessary;
},
child: AlertDialog(
shape: const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(10.0))),
title: Text(noticeUpdate[KEY_title],
style: const TextStyle(fontSize: 16)),
content: RichText(
text: TextSpan(
text: '${'현재버전'.tr} ${packageInfo.version} → ${'최신버전'.tr}',
style: const TextStyle(color: Colors.black87, fontSize: 12),
children: [
TextSpan(
text: latestVersion,
style: const TextStyle(
color: Colors.blue,
fontSize: 13,
fontWeight: FontWeight.bold))
]),
),
actions: [
if (!(isNecessary))
TextButton(
onPressed: () {
Get.back();
},
child: Text(
'취소'.tr,
style: const TextStyle(
color: Colors.grey,
),
))
else
TextButton(
onPressed: () {
SystemNavigator.pop();
},
child: Text(
'종료'.tr,
style: const TextStyle(
color: Colors.grey,
),
)),
TextButton(
onPressed: updateApp,
child: Text(
'업데이트'.tr,
style: const TextStyle(
color: Colors.blue, fontWeight: FontWeight.bold),
)),
],
),
),
barrierDismissible: !(isNecessary));
}
void updateApp() async {
String url = Platform.isAndroid ? androidStoreUrl : iosStoreUrl;
if (!await launchUrl(Uri.parse(url))) {
throw 'Could not launch $url';
}
}
Future<void> urgentDialog(Map<String, dynamic> noticeUrgentInspection,
PackageInfo packageInfo, String lastAllowVersion) async {
var isNecessary = noticeUrgentInspection[KEY_necessary] ?? false;
Get.dialog(
WillPopScope(
onWillPop: () async {
return !isNecessary;
},
child: AlertDialog(
shape: const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(10.0))),
title: Text(
noticeUrgentInspection[KEY_title],
style: const TextStyle(fontSize: 16),
),
content: Text(
noticeUrgentInspection[KEY_contents],
style: const TextStyle(fontSize: 14),
),
actions: [
TextButton(
onPressed: () async {
if (noticeUrgentInspection[KEY_kill_app] ?? false) {
SystemNavigator.pop();
} else {
Get.back();
}
},
child: const Text(
'확인',
style: TextStyle(
color: Colors.blue, fontWeight: FontWeight.bold),
)),
],
),
),
barrierDismissible: !(isNecessary));
}
}
728x90
'개발 > Flutter' 카테고리의 다른 글
flutter release version 에서 스크린샷만 보일 때 (0) | 2022.12.07 |
---|---|
How to get current route? 현재 라우트 받기 (0) | 2022.12.07 |
flutter fcm icon terminated FlutterLocalNotificationsPlugin (0) | 2022.12.03 |
instagram 같은 gridview를 원한다면 .. (0) | 2022.12.02 |
sliver_tool 사용해 보기 (0) | 2022.12.02 |